使用aspose-words库对word文档进行转换
介绍
Aspose.Words 是一种高级Word文档处理API,用于执行各种文档管理和操作任务。
API支持生成,修改,转换,呈现和打印文档,而无需在跨平台应用程序中直接使用Microsoft Word。
Aspose API支持流行文件格式处理,并允许将各类文档导出或转换为固定布局文件格式和最常用的图像/多媒体格式。
使用方法
引入依赖
aspose-words包不太好找,在阿里云镜像库中都没有,需要在网上下载后,上传到本地的私服库,或者用本地引入依赖的方式直接在lib中加载,下载地址。
将下载的jar包放到本地仓库中,路径为src/main/resources/lib/aspose-words-java-22.12-jdk17.jar
,在pom.xml中引入依赖,并配置plugin。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <dependency> <groupId>com.aspose</groupId> <artifactId>aspose-words</artifactId> <version>22.12</version> <scope>system</scope> <systemPath>${pom.basedir}/src/main/resources/lib/aspose-words-java-22.12-jdk17.jar</systemPath> </dependency>
<plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> <configuration> <includeSystemScope>true</includeSystemScope> </configuration> </plugin>
|
功能实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44
|
public class FileTransUtils {
public static void main(String[] args) throws Exception { String inPath = "/Users/zhyyao/Downloads/test2.docx"; String output = "/Users/zhyyao/Downloads/test2/test2.pdf"; doc2pdf(inPath, output); }
public static void doc2pdf(String inPath, String outPath) throws Exception { File file = new File(inPath);
FileInputStream fis = new FileInputStream(file); Document document = new Document(fis); if (!checkDirectory(outPath)) { throw new Exception("create dir failed"); } document.save(outPath, SaveFormat.PDF); }
public static boolean checkDirectory(String filePath) { File file = new File(filePath); if (file.isDirectory()) { return true; } else { File dir = file.getParentFile(); return dir == null || dir.isDirectory() || dir.mkdirs(); } }
}
|
局限性
此方法由于没有获取License,所以生成的pdf文件会带有水印。
如图所示:
获取License-去除水印
获取License
前往 Aspose 官网注册账户。
登录您的账户,然后在顶部导航栏中选择 “Pricing”(定价)选项。
在 “Pricing” 页面中,选择 “Purchase”(购买)选项。
您可以根据您的需求,选择适合的许可证类型和许可期限。然后点击 “BUY NOW”(立即购买)按钮。
接下来,您需要填写相关的购买信息,包括联系人信息、支付方式等。
完成付款后,您将收到购买确认和 License 文件。
将 License 文件添加到您的项目中。通常情况下,您需要将 License 文件作为资源添加到您的项目中,然后在代码中加载 License 文件。
配置License
在resources目录下创建license.xml文件,内容如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <License> <Data> <Products> <Product>Aspose.Total for Java</Product> <Product>Aspose.Words for Java</Product> </Products> <EditionType>Enterprise</EditionType> <SubscriptionExpiry>20991231</SubscriptionExpiry> <LicenseExpiry>20991231</LicenseExpiry> <SerialNumber>8bfe198c-7f0c-4ef8-8ff0-acc3237bf0d7</SerialNumber> </Data> <Signature> ****** </Signature> </License>
|
License认证
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| public static boolean getLicense() { boolean result = false; try { File file = ResourceUtils.getFile("classpath:license/license.xml"); FileInputStream inputStream = new FileInputStream(file); License aposeLic = new License(); aposeLic.setLicense(inputStream); result = true; } catch (Exception e) { e.printStackTrace(); } return result; }
public static void doc2pdf(String inPath, String outPath) throws Exception { if (!getLicense()) { return; }
File file = new File(inPath);
FileInputStream fis = new FileInputStream(file); Document document = new Document(fis); if (!checkDirectory(outPath)) { throw new Exception("create dir failed"); } document.save(outPath, SaveFormat.PDF); }
|
修改源码-去除水印
声明: 此方法仅供学习研究,请勿用于非法用途,需要商业版的请绕行,或请参考上一步。
⚠️ 注意: 本方法因为有部分脚本,需要在Linux或MacOs系统下运行。
准备源码jar包
- aspose-words版本最好为V22.12,其他版本可能略有不同,一下以V22.12为例。下载地址。
- javassist源码V3.29.2。Javassist(Java Programming Assistant)是一个用于操作 Java 字节码的库,允许在运行时动态修改类文件。 下载地址。
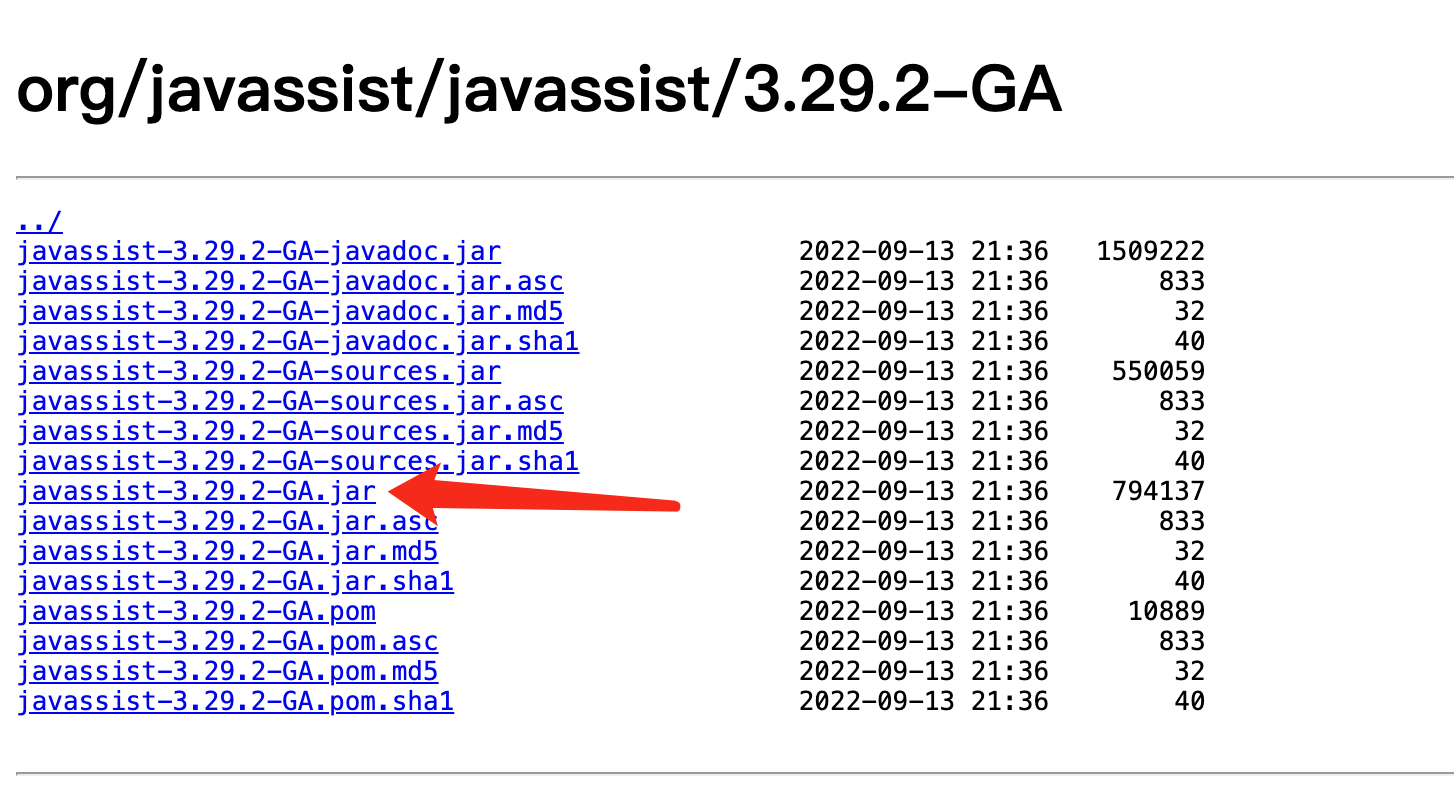
- java环境,不做描述。
添加配置文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| #!/bin/sh set -e SRC='aspose-words-22.12-jdk17.jar' DST='aspose-words-22.12-jdk17-cracked.jar' echo '==> 官网下载(新下载的此破解已失效,请直接下载破解版)' # stat $SRC || wget https://releases.aspose.com/java/repo/com/aspose/aspose-words/22.12/aspose-words-22.12-jdk17.jar echo '==> 重命名' rm -f $DST cp $SRC $DST echo '==> 执行破解,生成类' javac -classpath .:javassist-3.29.2-GA.jar AsposeWords_22_12.java java -classpath .:javassist-3.29.2-GA.jar AsposeWords_22_12 echo '==> 用jar命令更新jar内的类' echo '替换前' jar -uvf0 $DST com/aspose/words/*.class echo '==> 替换UCCESS。' echo '==> 用压缩工具打开,并删除 /MANIFEST.MF 目录下的*.SF,*.RSA文件,以及删除 versions 目录。' echo '==> DONE.'
|
- 添加修改文件(AsposeWords_22_12.java)
需注意:ClassPool.getDefault().insertClassPath("注意aspose-words-22.12-jdk17.jar路径");
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34
| import javassist.ClassPool; import javassist.CtClass; import javassist.CtMethod;
public class AsposeWords_22_12 { public static void main(String[] args) throws Exception { ClassPool.getDefault().insertClassPath("/Users/zhyyao/java/crack/aspose-words-22.12-jdk17.jar"); CtClass clazz = ClassPool.getDefault().getCtClass("com.aspose.words.zzYvW"); clazz.getDeclaredMethod("zzY34").setBody("{return com.aspose.words.zzZ8h.zzW8b;}"); clazz.getDeclaredMethod("zzjS").setBody("{this.zzWbe = com.aspose.words.zzZ8h.zzW8b; zzZAO = this; }"); CtMethod[] methodA = clazz.getDeclaredMethods(); for (CtMethod ctMethod : methodA) { CtClass[] ps = ctMethod.getParameterTypes(); if (ps.length == 1 && ctMethod.getName().equals("zzjS") && ps[0].getName().equals("java.io.InputStream")) { ctMethod.setBody("{this.zzWbe = com.aspose.words.zzZ8h.zzW8b; zzZAO = this; }"); } } clazz.getDeclaredConstructors()[0].setBody("{System.out.println(\"do nothing at zzYvW.\");}"); clazz.writeFile();
CtClass clazz2 = ClassPool.getDefault().getCtClass("com.aspose.words.zzWS8"); clazz2.getDeclaredMethod("zzQG").setBody("{System.out.println(\"do nothing. this.zzW67(); this.zzW5m();\");}"); clazz2.getDeclaredMethod("zzH0").setBody("{return com.aspose.words.zzY7H.zzZPG;}"); clazz2.writeFile();
CtClass clazz3 = ClassPool.getDefault().getCtClass("com.aspose.words.zzYJa"); clazz3.getDeclaredMethod("zzXuq").setBody("{zzYOl = 29273535023874148L;}"); clazz3.writeFile(); } }
|
将下载的源码放在指定目录,包括aspose-words-22.12-jdk17.jar和javassist-3.29.2-GA.jar。目录结构如下:
1 2 3 4 5
| crack/ ├── aspose-words-22.12-jdk17.jar ├── javassist-3.29.2-GA.jar ├── crack.sh └── AsposeWords_22_12.java
|
执行程序
执行crick.sh
执行成功后如下图所示,并生成aspose-words-22.12-jdk17-cracked.jar文件。
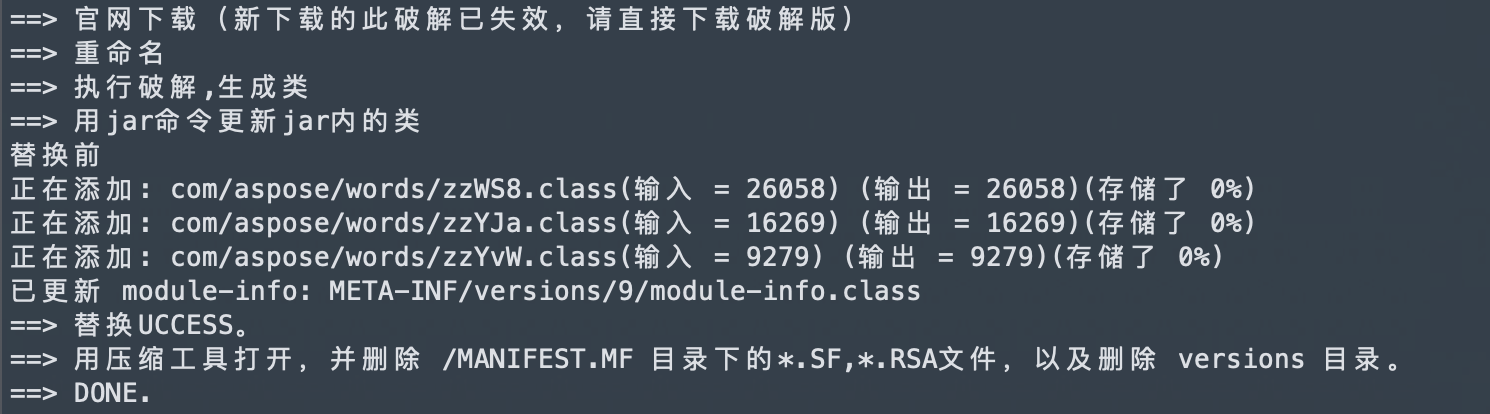
配置破解jar包
- 破解后的jar包因为有些验证hash的文件,所以不可以直接使用,需要先删除一些校验文件.
1 2 3 4 5 6 7
| cd crack mkdir extracted cp aspose-words-22.12-jdk17-cracked.jar extracted/ cd extracted jar xf aspose-words-22.12-jdk17-cracked.jar rm aspose-words-22.12-jdk17-cracked.jar rm -rf META-INF/**
|
- 在META-INF目录下重新添加自定义的MANIFEST.MF文件。
注意:文末有空行。
1 2 3 4 5 6 7 8 9 10
| Manifest-Version: 1.0 Application-Name: Aspose.Words for Java Implementation-Title: Aspose.Words for Java Bundle-SymbolicName: com.aspose.words Implementation-Version: 22.12.0 Release-Date: 2022.12.01 Specification-Vendor: Aspose Pty Ltd Bundle-ManifestVersion: 2 Created-By: 17.0.7 (Oracle Corporation)
|
1 2 3 4
| cd crack/extracted mkdir test mv com test jar cfm cracked.jar META-INF/MANIFEST.MF -C test/ .
|
打包之后产生的cracked.jar文件就是破解后的jar包。
测试验证
1 2 3 4 5 6 7 8
| <dependency> <groupId>com.aspose</groupId> <artifactId>aspose-words</artifactId> <version>22.12</version> <scope>system</scope> <systemPath>${pom.basedir}/src/main/resources/lib/cracked/cracked.jar</systemPath> </dependency>
|
执行mvn clean && mvn install && mvn compile
重新执行代码,doc2pdf(..,..) 函数,执行如图所示,水印已成功去除。
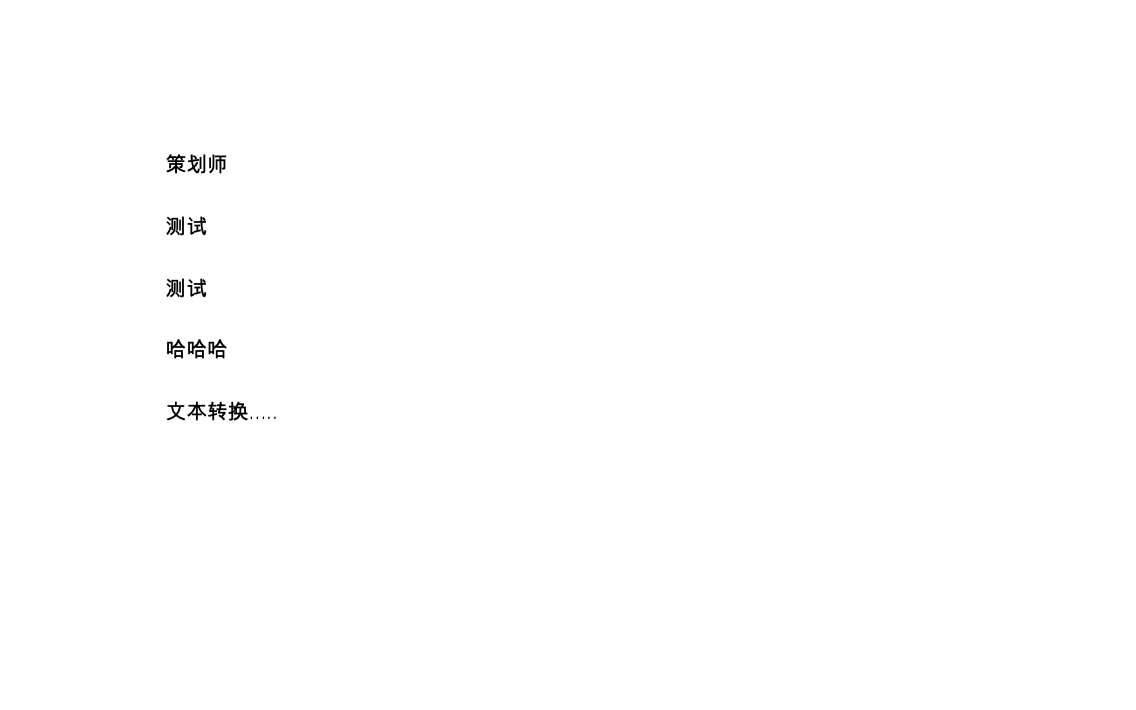